# Asky
> Ansi + ask + yes = Asky
Good looking prompts for the terminal.
## Usage
First of all, this is a library, so you need to add this to your project
```bash
cargo add asky
```
Then, you can [see the documentation](https://docs.rs/asky/).
## Demos
### Confirm
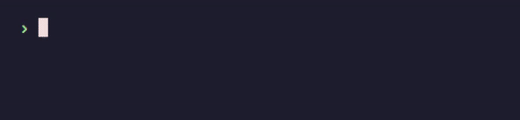
Code:
```rust
use asky::Confirm;
fn main() -> std::io::Result<()> {
if Confirm::new("Do you like coffe?").prompt()? {
println!("Great, me too!");
}
// ...
Ok(())
}
```
### Toggle
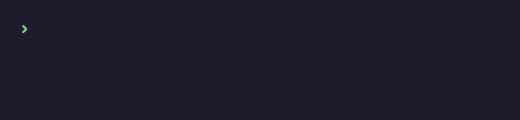
Code:
```rust
use asky::Toggle;
fn main() -> std::io::Result<()> {
let tabs = Toggle::new("Which is better?", ["Tabs", "Spaces"]).prompt()?;
println!("Great choice");
// ...
Ok(())
}
```
### Text
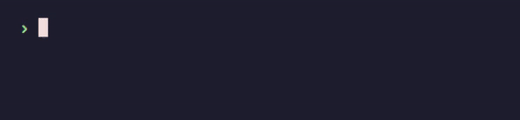
Code:
```rust
use asky::Text;
fn main() -> std::io::Result<()> {
let color = Text::new("What's your favorite color?").prompt()?;
println!("{color} is a beautiful color");
// ...
Ok(())
}
```
### Number
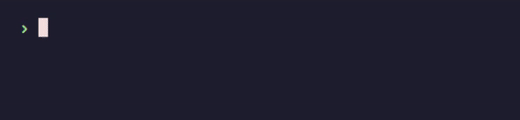
Code:
```rust
use asky::Number;
fn main() -> std::io::Result<()> {
let age: = Number::::new("How old are you?").prompt()?;
if let Ok(age) = Number::::new("How old are you?").prompt()? {
if age <= 60 {
println!("Pretty young");
}
}
// ...
Ok(())
}
```
### Password
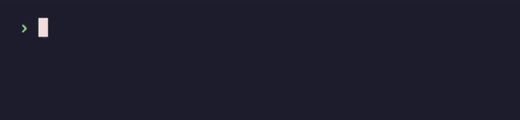
Code:
```rust
use asky::Password;
fn main() -> std::io::Result<()> {
let password = Password::new("What's your IG password?").prompt()?;
if password.len() >= 1 {
println!("Ultra secure!");
}
// ...
Ok(())
}
```
### Select
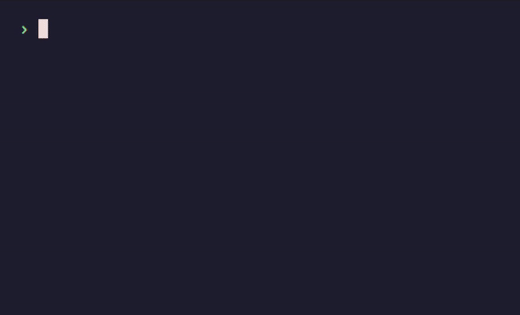
Code:
```rust
use asky::Select;
fn main() -> std::io::Result<()> {
let choice = Select::new("Choose number", 1..=30).prompt()?;
println!("{choice}, Interesting choice");
// ...
Ok(())
}
```
### MultiSelect
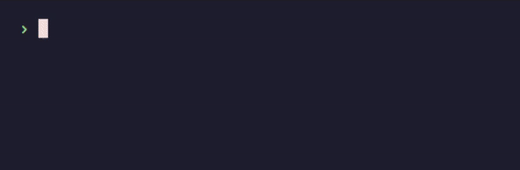
Code:
```rust
use asky::MultiSelect;
fn main() -> std::io::Result<()> {
let opts = ["Dog", "Cat", "Fish", "Bird", "Other"];
let choices = MultiSelect::new("What kind of pets do you have?", opts).prompt()?;
if choices.len() > 2 {
println!("So you love pets");
}
// ...
Ok(())
}
```
## Mentions
Inspired by:
- [Prompts](https://www.npmjs.com/package/prompts) - Lightweight, beautiful and user-friendly interactive prompts
- [Astro](https://astro.build/) - All-in-one web framework with a beautiful command line tool
- [Gum](https://github.com/charmbracelet/gum) - A tool for glamorous shell scripts
Alternatives:
- [Dialoguer](https://github.com/console-rs/dialoguer) - A command line prompting library.
- [Inquire](https://github.com/mikaelmello/inquire) - A library for building interactive prompts on terminals.
- [Requestty](https://github.com/Lutetium-Vanadium/requestty) - An easy-to-use collection of interactive cli prompts.
---
License: [MIT](LICENSE)