# Riichi Mahjong Calculator Via the Terminal
CLI tool that calculates the score of a hand in riichi mahjong.
- Manual mode (Calculator Mode): given han and fu, calculates the score
- Normal mode: given a hand, calculates the score with included yaku and fu
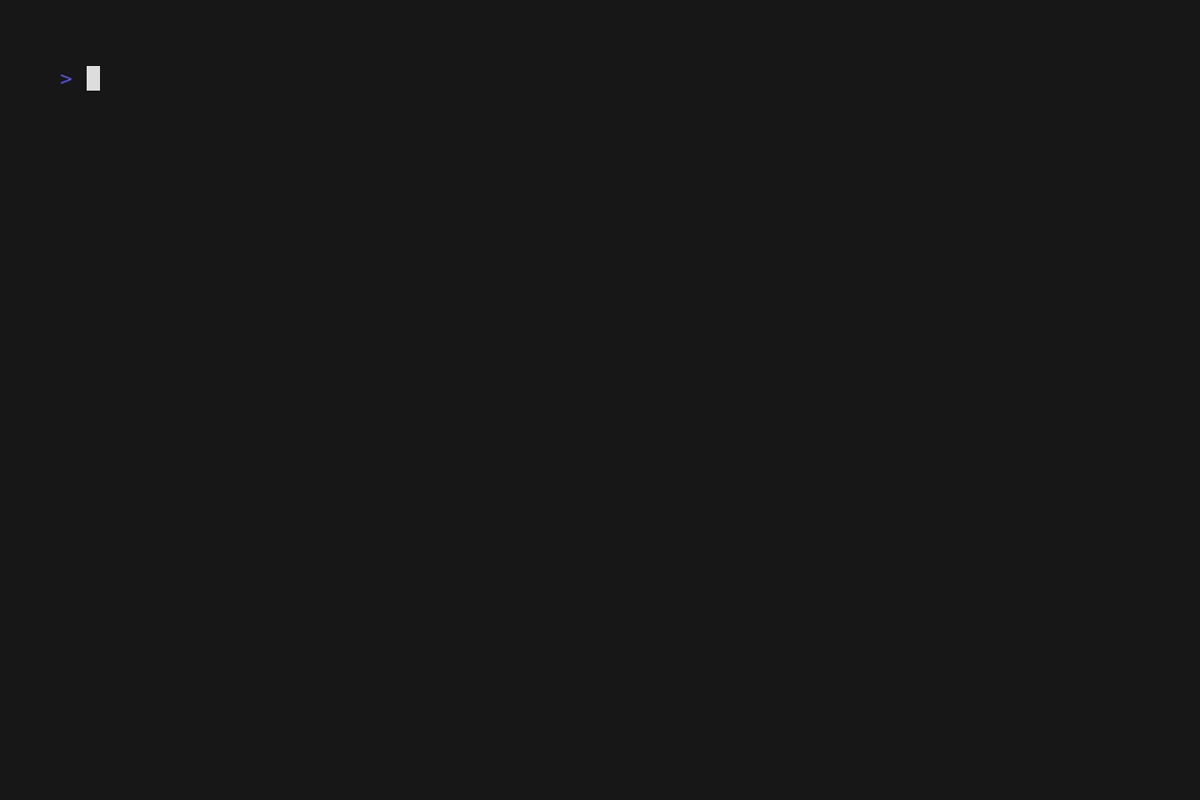
## Examples
### Calculator Mode
```bash
~/$ mahc -m 4 30 --ba 3
> Dealer: 12500 (4200)
non-dealer: 8600 (2300/ 4200)
```
### Normal Mode
note: the winning group has to go last (this is to calculate fu correctly)
``` bash
~/$ mahc --tiles 777z 111z 234p 234p 11p -w 1p -p Ew -s Ew
> 7 Han/ 50 Fu
Dealer: 18000 (6000)
Non-dealer: 12000 (3000/6000)
Yaku:
Iipeikou: 1
Honitsu: 3
Yakuhai: 1
Yakuhai: 1
Yakuhai: 1
Fu:
BasePoints: 20
ClosedRon: 10
NonSimpleClosedTriplet: 8
NonSimpleClosedTriplet: 8
SingleWait: 2
```
### Using file input
```
# hands.txt
--tiles 1p 9p 1s 9s 1m 9m rd gd wd Ew Sw Nw WWw -w Ww -p Ew -s Ew
--tiles 11z NNw SSw WWw rrd wwd ggd -w gd -p Ew -s Ew -d Ew Ew
-m 4 30 --ba 3
```
```bash
~/$ mahc -f hands.txt
❯ Dealer: 96000 (32000)
Non-dealer: 64000 (16000/32000)
Yaku:
KokushiMusou Yakuman
KokushiMusou Yakuman 13 sided wait
Dealer: 144000 (48000)
Non-dealer: 96000 (24000/48000)
Yaku:
Tsuuiisou Yakuman
Daichiishin Yakuman
Shousuushii Yakuman
4 Han/ 30 Fu/ 3 Honba
Dealer: 12500 (4200)
non-dealer: 8600 (2300/4200)
```
### Json out
in ***normal mode***
```bash
~/$ mahc --tiles 123p 456p 789p rrrdo 99p -w 9p -p Ew -s Ew -d 9p --json
```
yields
```json
{
"dora":1,
"fu":30,
"fuString":[ "BasePoints: 20", "NonSimpleOpenTriplet: 4", "SingleWait: 2"
],
"han":5,
"honba":0,
"scores":{
"dealer":{"ron":12000,"tsumo":4000},
"non-dealer":{
"ron":8000,
"tsumo":{"dealer":4000,"non-dealer":2000}
}
},
"yakuString":["Honitsu: 2","Ittsuu: 1","Yakuhai: 1"]}
```
and in ***calculator mode***
```bash
~/$ mahc -m 4 30 --ba 3 --json
```
yields
```json
{
"fu":30,
"han":4,
"honba":3,
"scores":{
"dealer":{ "ron":12500, "tsumo":4200 },
"non-dealer":{ "ron":8600, "tsumo":{ "dealer":4200, "non-dealer":2300 }
}
}
}
```
## Notation
### Suits
| Type | Notation |
|-------|-----------------------------------------------|
| Man (Characters) | 1m, 2m, 3m, 4m, 5m, 6m, 7m, 8m, 9m |
| Pin (Circles) | 1p, 2p, 3p, 4p, 5p, 6p, 7p, 8p, 9p |
| Sou (Bamboos) | 1s, 2s, 3s, 4s, 5s, 6s, 7s, 8s, 9s |
### Honors
| Type | Notation | MPSZ Notation |
|---------|----------------|--------------------|
| Winds | Ew, Sw, Ww, Nw |1z, 2z, 3z, 4z |
| Dragons | wd, gd, rd |5z, 6z, 7z |
### Special Notation
| Description | Example |
|-----------------|-------------------------------------------------|
| Open Sets | 234po (an open sequence of 2, 3, 4 in Pin suit) |
| akadora | 0m, 0p, 0s |
- eg: EEEw (triplet of east wind)
- eg: 234m (sequence of 2 3 4 Man)
- eg: 406s (sequence of 4 5 6 Sou with the akadora 5 sou)
- eg: rrrrdo (open quad of red dragon)
- eg: 11s (pair of 1 sou)
- eg: 8m (8 man tile)
## Installation
#### *using cargo*
```
cargo install mahc
mahc --version
```
#### *build from source*
```
git clone https://github.com/DrCheeseFace/mahc
cd mahc
cargo build
./target/debug/mahc --version
```
#### *from latest release*
```
curl -s https://api.github.com/repos/DrCheeseFace/rusty-riichi-mahjong-calculator/releases/latest | grep "browser_download_url" | cut -d '"' -f 4 | wget -i -
unzip mahc-v1.1.0-x86_64-unknown-linux-gnu.zip -d mahc
cd mahc/x86_64-unknown-linux-gnu/release
./mahc --version
```
## Implemented hand validations as of yet
##### One Han Yaku
- [x] Tanyao
- [x] Iipeikou
- [x] Yakuhai
- [x] MenzenTsumo
- [x] Pinfu
- [x] Riichi
- [x] Ippatsu
- [x] Haitei
- [x] RinshanKaihou
- [x] Chankan
##### Two Han Yaku
- [x] DoubleRiichi
- [x] Toitoi
- [x] Ittsuu
- [x] SanshokuDoujun
- [x] Chantaiyao
- [x] Sanankou
- [x] SanshokuDoukou
- [x] Sankantsu
- [x] Honroutou
- [x] Shousangen
- [x] Chiitoitsu
##### Three Han Yaku
- [x] Honitsu
- [x] JunchanTaiyao
- [x] Ryanpeikou
##### Six Han Yaku
- [x] Chinitsu
##### Yakuman
- [x] KazoeYakuman
- [x] KokushiMusou
- [x] KokushiMusou 13 sided wait
- [x] Suuankou
- [x] Suuankou tanki wait
- [x] Daisangen
- [x] Shousuushii
- [x] Daisuushii
- [x] Tsuuiisou
- [x] Daiichishin
- [x] Chinroutou
- [x] Ryuuiisou
- [x] ChuurenPoutou
- [x] ChuurenPoutou 9 sided wait
- [x] Suukantsu
- [x] Tenhou
- [x] Chiihou
## TODO
- [x] validation a hand is possible (eg not having 20 east tiles :)
- [x] add all da yaku
- [x] validation on if yaku is there
- [x] validate winning tile
- [x] propogate the errors up for a nice printout
- [x] validate stuff like cant riichi and double riichi. all that haitei, chankan rinshan shizz
- [x] file stdIn
- [x] json out flag
- [x] tile input for ```--dora``` flag
- [ ] add more comprehensive tests
- [ ] document the undocumented
## Contributing
- If you spot a bug (which there probabably are many), put in an issue with how to reproduce it
- if youd like to contribute, DO IT (send a PR)
- keep in mind, we pretty far from finishing it currently. so FUCK validation (for the time being)
